Picture this scenario:
You have a ServiceNow application that has multiple database tables that support a specific business process. In 2 of those tables you have After Business Rules that run the same code that creates a new record in another of the application’s tables. That may look like this:
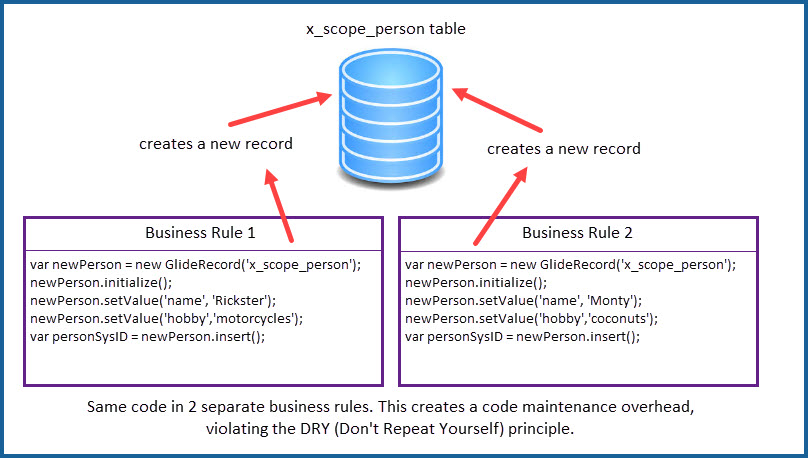
In the above situation we have the same code creating a new x_scope_person record in 2 separate places. This means if we need to change that code for any reason, we need to update the code in 2 separate places. Now imagine you have 10 business rules that all use the same code to create this x_scope_person record–that would be a nightmare to maintain!
Having the same code in multiple places violates the DRY principle (Don’t Repeat Yourself).
The solution is to create a Script Include and create a method to create a new x_scope_person record. That method can accept information that represents the fields and associated values to use.
So in the script include we may have a method called createPersonRecord(). That method could accept an object that contains the field name and field values we’ll use to create that new Person record. That code may look like this:
// NOTE: this Script Include is named PersonUtil()
createNewPerson: function(personObj){
var newPerson = new GlideRecord('x_scope_person');
newPerson.initialize();
newPerson.setValue('active','true');
// cycle through the personObj and assign values to fields
for (var fieldName in personObj) {
var fieldValue = personObj[fieldName];
newPerson.setValue(fieldName, fieldValue);
}
var newPersonSysID = newPerson.insert();
return newPersonSysID;
},
Now, the code in each of those business rules will call that method in the Script Include named PersonUtil() and pass in an object containing the field name and values for each field name.
// Business Rule 1
var personObj = {
'first_name':'Rick',
'last_name': 'McGargles',
'hobby':'Motorcycles',
'favorite_bird':'African Swallow'
};
var personUtil = new PersonUtil();
var newPersonSysID = personUtil.createNewPerson(personObj);
// Business Rule 2
var personObj = {
'first_name':'Monty',
'last_name':'Python',
'hobby':'Clacking Coconuts',
'favorite_bird':'European Swallow'
};
var personUtil = new PersonUtil();
var newPersonSysID = personUtil.createNewPerson(personObj);
So what is a Script Include?
Think of a script include as a library of reusable code that we can use to do things from multiple code places in our application. Script includes typically have a series of methods that are grouped based on some commonality. In the above Script Include there would be a series of methods that interact with the Person table–getting data, creating records, updating records, etc.
And why would we want a Script Include?
- They reduce code maintenance overhead
- They help us adhere to the DRY principle
- It’s what all the ServiceNow cool cats do so you have something to talk about at developer parties